- 44
- 101
- Версия SA-MP
-
- 0.3.7 (R1)
- 0.3.7-R2
- 0.3.7-R3
- 0.3.7-R4
- 0.3DL
- 0.3e (R1) / CR-MP
- CR-MP 0.3.7
- Любая
- Другая
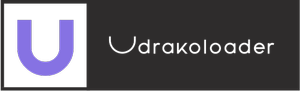
Udrakoloader is a mod for GTA San Andreas. Provides the ability to load scripts (DLL) developed in .Net into the game.
The loader is not limited to just GTA SA, it also works on HALO CE and PC and maybe many other games like GTA 5 with the ASI Loader.
Based on ScriptHookvDotNet and SharpNeedle.
Source Code : https://github.com/DestroyerDarkNess/Udrakoloader
Some examples : [Compile DLL and put inside the "Udrakoloader" folder]
For C # Plugins, you must provide the correct Namespace directory.
In this case Replace "namespace ExampleProject.Udrakoloader" with "namespace <Your Root Namespace Project>.Udrakoloader"
UdrakoLoader C# Plugin Example:
// Plugin.cs
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace ExampleProject.Udrakoloader
{
public class Plugin
{
public static int EntryPoint(string pwzArgument)
{
string processName = Process.GetCurrentProcess().ProcessName;
MessageBox.Show("C# Example | The current process is " + processName);
return 0;
}
}
}
UdrakoLoader VB Plugin Example:
'Plugin.vb
Imports System
Imports System.Collections.Generic
Imports System.Diagnostics
Imports System.Linq
Imports System.Text
Imports System.Windows.Forms
Imports System.Reflection
Imports System.Runtime.CompilerServices
Imports System.Runtime.InteropServices
Imports System.Security
Namespace Udrakoloader
Public Class Plugin
Public Shared Function EntryPoint(ByVal pwzArgument As String) As Integer
Dim processName As String = Process.GetCurrentProcess().ProcessName
MessageBox.Show("vb Example | The current process is " & processName)
Return 0
End Function
End Class
End Namespace
Attention:
- The .net DLLs must be compiled under the .Net Framework 4.0.
- The name of the Plugin DLL must be the same as the Root Namespace.
Requirements.
It has not been tested on 64bits. You can contact me by discord: Destroyer#8328
Wrapper for the use of Open SAMP API :
To work with OPEN SAMP API, you must add the references to the project:- System.Text
- System.Drawing
- System.Windows.Forms (this reference is added Optional)
Open SAMP API - C# Wrapper:
//API.cs
//API.cs
using System;
using System.Drawing;
using System.Runtime.InteropServices;
using System.Drawing;
using System.Text;
namespace SAMP_API
{
class API
{
public const String PATH = "\\lib\\Open-SAMP-API.dll";
// Client.hpp
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int Init();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern void SetParam(string _szParamName, string _szParamValue);
// GTAFunctions.hpp
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetGTACommandLine(ref StringBuilder line, int max_len);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern bool IsMenuOpen();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern bool ScreenToWorld(float x, float y, out float worldX, out float worldY, out float worldZ);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern bool WorldToScreen(float x, float y, float z, out float screenX, out float screenY);
// PlayerFunctions.hpp
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerCPed();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerHealth();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerArmor();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerMoney();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerSkinID();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerInterior();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsPlayerInAnyVehicle();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsPlayerDriver();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsPlayerPassenger();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsPlayerInInterior();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerX(out float posX);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerY(out float posY);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerZ(out float posZ);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerPosition(out float posX, out float posY, out float posZ);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsPlayerInRange2D(float posX, float posY, float radius);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsPlayerInRange3D(float posX, float posY, float posZ, float radius);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetCityName(ref StringBuilder cityName, int max_len);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetZoneName(ref StringBuilder zoneName, int max_len);
// RenderFunctions.hpp
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int TextCreate(string Font, int FontSize, bool bBold, bool bItalic, int x, int y, int color, string text, bool bShadow, bool bShow);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int TextDestroy(int ID);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int TextSetShadow(int id, bool b);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int TextSetShown(int id, bool b);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int TextSetColor(int id, int color);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int TextSetPos(int id, int x, int y);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int TextSetString(int id, string str);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int TextUpdate(int id, string Font, int FontSize, bool bBold, bool bItalic);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int BoxCreate(int x, int y, int w, int h, int dwColor, bool bShow);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int BoxDestroy(int id);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int BoxSetShown(int id, bool bShown);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int BoxSetBorder(int id, int height, bool bShown);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int BoxSetBorderColor(int id, int dwColor);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int BoxSetColor(int id, int dwColor);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int BoxSetHeight(int id, int height);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int BoxSetPos(int id, int x, int y);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int BoxSetWidth(int id, int width);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int LineCreate(int x1, int y1, int x2, int y2, int width, int color, bool bShow);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int LineDestroy(int id);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int LineSetShown(int id, bool bShown);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int LineSetColor(int id, int color);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int LineSetWidth(int id, int width);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int LineSetPos(int id, int x1, int y1, int x2, int y2);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int ImageCreate(string path, int x, int y, int rotation, int align, bool bShow);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int ImageDestroy(int id);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int ImageSetShown(int id, bool bShown);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int ImageSetAlign(int id, int align);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int ImageSetPos(int id, int x, int y);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int ImageSetRotation(int id, int rotation);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int DestroyAllVisual();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int ShowAllVisual();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int HideAllVisual();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetFrameRate();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetScreenSpecs(out int width, out int height);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int SetCalculationRatio(int width, int height);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int SetOverlayPriority(int id, int priority);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int SetOverlayCalculationEnabled(int id, bool enabled);
// SAMPFunctions.hpp
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetServerIP(ref StringBuilder ip, int max_len);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetServerPort();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int SendChat(string msg);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int ShowGameText(string msg, int time, int style);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int AddChatMessage(string msg);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int ShowDialog(int id, int style, string caption, string text, string button, string button2);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerNameByID(int id, ref StringBuilder playername, int max_len);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerIDByName(string name);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerName(ref StringBuilder playername, int max_len);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerId();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsChatOpen();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsDialogOpen();
// VehicleFunctions.hpp
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetVehiclePointer();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetVehicleSpeed(float factor);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern float GetVehicleHealth();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetVehicleModelId();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetVehicleModelName(ref StringBuilder name, int max_len);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetVehicleModelNameById(int vehicleID, ref StringBuilder name, int max_len);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetVehicleType();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetVehicleFreeSeats(out int seatFL, out int seatFR, out int seatRL, out int seatRR);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetVehicleFirstColor();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetVehicleSecondColor();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetVehicleColor(out int color1, out int color2);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehicleSeatUsed(int seat);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehicleLocked();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehicleHornEnabled();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehicleSirenEnabled();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehicleAlternateSirenEnabled();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehicleEngineEnabled();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehicleLightEnabled();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehicleCar();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehiclePlane();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehicleBoat();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehicleTrain();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int IsVehicleBike();
// WeaponFunctions.hpp
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int HasWeaponIDClip(int weaponID);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerWeaponID();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerWeaponType();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerWeaponSlot();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerWeaponName(int dwWeapSlot, ref StringBuilder _szWeapName, int max_len);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerWeaponClip(int dwWeapSlot);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerWeaponTotalClip(int dwWeapSlot);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerWeaponState();
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerWeaponAmmo(int weaponType);
[DllImport(PATH, CallingConvention = CallingConvention.Cdecl)]
public static extern int GetPlayerWeaponAmmoInClip(int weaponType);
/// <summary>
/// Send a message/command to the server
/// </summary>
/// <param name="message">The message/command</param>
/// <param name="args">Arguments for a command, e.g an ID</param>
public static void SendChatEx(string message, params object[] args)
{
if (message.Length != 0)
{
if (args.Length > 0)
message += " " + string.Join(" ", args);
SendChat(message);
}
}
/// <summary>
/// Add a new message in the SAMP chat (only local)
/// </summary>
/// <param name="text">The text to be written</param>
/// <param name="color">A color in Hex</param>
public static void AddChatMessageEx(string text, string color = "FFFFFF")
{
AddChatMessage("{" + color + "}" + text);
}
/// <summary>
/// Add a new message in the SAMP chat (only local)
/// </summary>
/// <param name="text">The text to be written</param>
/// <param name="color">A Color-Type</param>
public static void AddChatMessageEx(string text, Color color)
{
AddChatMessage("{" + ColorToHexRGB(color) + "}" + text);
}
/// <summary>
/// Add a new message in the SAMP chat (only local)
/// </summary>
/// <param name="prefix">The prefix to be written</param>
/// <param name="prefixColor">A prefix color in Hex</param>
/// <param name="text">The text to be written</param>
/// <param name="color">A color in Hex</param>
public static void AddChatMessageEx(string prefix, string prefixColor, string text, string color = "FFFFFF")
{
AddChatMessage("{" + prefixColor + "}" + prefix + " {" + color + "}" + text);
}
/// <summary>
/// Add a new message in the SAMP chat (only local)
/// </summary>
/// <param name="prefix">The prefix to be written</param>
/// <param name="prefixColor">A Color-Type</param>
/// <param name="text">The text to be written</param>
/// <param name="color">A Color-Type</param>
public static void AddChatMessageEx(string prefix, Color prefixColor, string text, Color color)
{
AddChatMessage("{" + ColorToHexRGB(prefixColor) + "}" + prefix + " {" + ColorToHexRGB(color) + "}" + text);
}
public static string GetPlayerNameByIDEx(int id)
{
StringBuilder builder = new StringBuilder(32);
GetPlayerNameByID(id, ref builder, builder.Capacity);
return builder.ToString();
}
/// <summary>
/// Convert a Color to Hex (RGB)
/// </summary>
/// <param name="color">Color convert to Hex</param>
/// <returns></returns>
public static String ColorToHexRGB(Color color)
{
return color.R.ToString("X2") + color.G.ToString("X2") + color.B.ToString("X2");
}
/// <summary>
/// Convert a Color to Hex (ARGB)
/// </summary>
/// <param name="color">Color convert to Hex</param>
/// <returns></returns>
public static String ColorToHexARGB(Color color)
{
return color.A.ToString("X2") + color.R.ToString("X2") + color.G.ToString("X2") + color.B.ToString("X2");
}
}
}
Open SAMP API - VB Wrapper:
Imports System
Imports System.Drawing
Imports System.Runtime.InteropServices
Imports System.Text
Namespace SAMP_API
Class API
Public Const PATH As String = "\lib\Open-SAMP-API.dll"
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function Init() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Sub SetParam(ByVal _szParamName As String, ByVal _szParamValue As String)
End Sub
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetGTACommandLine(ByRef line As StringBuilder, ByVal max_len As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsMenuOpen() As Boolean
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function ScreenToWorld(ByVal x As Single, ByVal y As Single, <Out> ByRef worldX As Single, <Out> ByRef worldY As Single, <Out> ByRef worldZ As Single) As Boolean
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function WorldToScreen(ByVal x As Single, ByVal y As Single, ByVal z As Single, <Out> ByRef screenX As Single, <Out> ByRef screenY As Single) As Boolean
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerCPed() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerHealth() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerArmor() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerMoney() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerSkinID() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerInterior() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsPlayerInAnyVehicle() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsPlayerDriver() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsPlayerPassenger() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsPlayerInInterior() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerX(<Out> ByRef posX As Single) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerY(<Out> ByRef posY As Single) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerZ(<Out> ByRef posZ As Single) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerPosition(<Out> ByRef posX As Single, <Out> ByRef posY As Single, <Out> ByRef posZ As Single) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsPlayerInRange2D(ByVal posX As Single, ByVal posY As Single, ByVal radius As Single) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsPlayerInRange3D(ByVal posX As Single, ByVal posY As Single, ByVal posZ As Single, ByVal radius As Single) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetCityName(ByRef cityName As StringBuilder, ByVal max_len As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetZoneName(ByRef zoneName As StringBuilder, ByVal max_len As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function TextCreate(ByVal Font As String, ByVal FontSize As Integer, ByVal bBold As Boolean, ByVal bItalic As Boolean, ByVal x As Integer, ByVal y As Integer, ByVal color As Integer, ByVal text As String, ByVal bShadow As Boolean, ByVal bShow As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function TextDestroy(ByVal ID As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function TextSetShadow(ByVal id As Integer, ByVal b As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function TextSetShown(ByVal id As Integer, ByVal b As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function TextSetColor(ByVal id As Integer, ByVal color As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function TextSetPos(ByVal id As Integer, ByVal x As Integer, ByVal y As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function TextSetString(ByVal id As Integer, ByVal str As String) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function TextUpdate(ByVal id As Integer, ByVal Font As String, ByVal FontSize As Integer, ByVal bBold As Boolean, ByVal bItalic As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function BoxCreate(ByVal x As Integer, ByVal y As Integer, ByVal w As Integer, ByVal h As Integer, ByVal dwColor As Integer, ByVal bShow As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function BoxDestroy(ByVal id As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function BoxSetShown(ByVal id As Integer, ByVal bShown As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function BoxSetBorder(ByVal id As Integer, ByVal height As Integer, ByVal bShown As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function BoxSetBorderColor(ByVal id As Integer, ByVal dwColor As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function BoxSetColor(ByVal id As Integer, ByVal dwColor As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function BoxSetHeight(ByVal id As Integer, ByVal height As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function BoxSetPos(ByVal id As Integer, ByVal x As Integer, ByVal y As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function BoxSetWidth(ByVal id As Integer, ByVal width As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function LineCreate(ByVal x1 As Integer, ByVal y1 As Integer, ByVal x2 As Integer, ByVal y2 As Integer, ByVal width As Integer, ByVal color As Integer, ByVal bShow As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function LineDestroy(ByVal id As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function LineSetShown(ByVal id As Integer, ByVal bShown As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function LineSetColor(ByVal id As Integer, ByVal color As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function LineSetWidth(ByVal id As Integer, ByVal width As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function LineSetPos(ByVal id As Integer, ByVal x1 As Integer, ByVal y1 As Integer, ByVal x2 As Integer, ByVal y2 As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function ImageCreate(ByVal path As String, ByVal x As Integer, ByVal y As Integer, ByVal rotation As Integer, ByVal align As Integer, ByVal bShow As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function ImageDestroy(ByVal id As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function ImageSetShown(ByVal id As Integer, ByVal bShown As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function ImageSetAlign(ByVal id As Integer, ByVal align As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function ImageSetPos(ByVal id As Integer, ByVal x As Integer, ByVal y As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function ImageSetRotation(ByVal id As Integer, ByVal rotation As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function DestroyAllVisual() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function ShowAllVisual() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function HideAllVisual() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetFrameRate() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetScreenSpecs(<Out> ByRef width As Integer, <Out> ByRef height As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function SetCalculationRatio(ByVal width As Integer, ByVal height As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function SetOverlayPriority(ByVal id As Integer, ByVal priority As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function SetOverlayCalculationEnabled(ByVal id As Integer, ByVal enabled As Boolean) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetServerIP(ByRef ip As StringBuilder, ByVal max_len As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetServerPort() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function SendChat(ByVal msg As String) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function ShowGameText(ByVal msg As String, ByVal time As Integer, ByVal style As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function AddChatMessage(ByVal msg As String) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function ShowDialog(ByVal id As Integer, ByVal style As Integer, ByVal caption As String, ByVal text As String, ByVal button As String, ByVal button2 As String) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerNameByID(ByVal id As Integer, ByRef playername As StringBuilder, ByVal max_len As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerIDByName(ByVal name As String) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerName(ByRef playername As StringBuilder, ByVal max_len As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerId() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsChatOpen() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsDialogOpen() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetVehiclePointer() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetVehicleSpeed(ByVal factor As Single) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetVehicleHealth() As Single
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetVehicleModelId() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetVehicleModelName(ByRef name As StringBuilder, ByVal max_len As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetVehicleModelNameById(ByVal vehicleID As Integer, ByRef name As StringBuilder, ByVal max_len As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetVehicleType() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetVehicleFreeSeats(<Out> ByRef seatFL As Integer, <Out> ByRef seatFR As Integer, <Out> ByRef seatRL As Integer, <Out> ByRef seatRR As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetVehicleFirstColor() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetVehicleSecondColor() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetVehicleColor(<Out> ByRef color1 As Integer, <Out> ByRef color2 As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehicleSeatUsed(ByVal seat As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehicleLocked() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehicleHornEnabled() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehicleSirenEnabled() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehicleAlternateSirenEnabled() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehicleEngineEnabled() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehicleLightEnabled() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehicleCar() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehiclePlane() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehicleBoat() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehicleTrain() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function IsVehicleBike() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function HasWeaponIDClip(ByVal weaponID As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerWeaponID() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerWeaponType() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerWeaponSlot() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerWeaponName(ByVal dwWeapSlot As Integer, ByRef _szWeapName As StringBuilder, ByVal max_len As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerWeaponClip(ByVal dwWeapSlot As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerWeaponTotalClip(ByVal dwWeapSlot As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerWeaponState() As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerWeaponAmmo(ByVal weaponType As Integer) As Integer
End Function
<DllImport(PATH, CallingConvention:=CallingConvention.Cdecl)>
Public Shared Function GetPlayerWeaponAmmoInClip(ByVal weaponType As Integer) As Integer
End Function
Public Shared Sub SendChatEx(ByVal message As String, ParamArray args As Object())
If message.Length <> 0 Then
If args.Length > 0 Then message += " " & String.Join(" ", args)
SendChat(message)
End If
End Sub
Public Shared Sub AddChatMessageEx(ByVal text As String, Optional ByVal color As String = "FFFFFF")
AddChatMessage("{" & color & "}" & text)
End Sub
Public Shared Sub AddChatMessageEx(ByVal text As String, ByVal color As Color)
AddChatMessage("{" & ColorToHexRGB(color) & "}" & text)
End Sub
Public Shared Sub AddChatMessageEx(ByVal prefix As String, ByVal prefixColor As String, ByVal text As String, Optional ByVal color As String = "FFFFFF")
AddChatMessage("{" & prefixColor & "}" & prefix & " {" & color & "}" & text)
End Sub
Public Shared Sub AddChatMessageEx(ByVal prefix As String, ByVal prefixColor As Color, ByVal text As String, ByVal color As Color)
AddChatMessage("{" & ColorToHexRGB(prefixColor) & "}" & prefix & " {" & ColorToHexRGB(color) & "}" & text)
End Sub
Public Shared Function GetPlayerNameByIDEx(ByVal id As Integer) As String
Dim builder As StringBuilder = New StringBuilder(32)
GetPlayerNameByID(id, builder, builder.Capacity)
Return builder.ToString()
End Function
Public Shared Function ColorToHexRGB(ByVal color As Color) As String
Return color.R.ToString("X2") + color.G.ToString("X2") + color.B.ToString("X2")
End Function
Public Shared Function ColorToHexARGB(ByVal color As Color) As String
Return color.A.ToString("X2") + color.R.ToString("X2") + color.G.ToString("X2") + color.B.ToString("X2")
End Function
Public Shared Function colorFormat(ByVal c As Color) As Integer
Return c.ToArgb
End Function
Public Shared Function GetScreenSpecsOverlay() As Point
Dim x As Integer
Dim y As Integer
GetScreenSpecs(x, y)
Return New Point(x, y)
End Function
End Class
End Namespace
Plugin Example :
SAMP DLL Injector
Injector For SAMP [San Andreas Multi-Player] | Plugin For Udrakoloader .
Source Code : https://github.com/DestroyerDarkNess/SAMP-Dll-Injector
Instructions- Extract into the "Udrakoloader" folder.
- Put Your DLL to Inject in the folder [SAMPInjector] and Enjoy.
Вложения
Последнее редактирование: